How To Print Html To Pdf In angular.
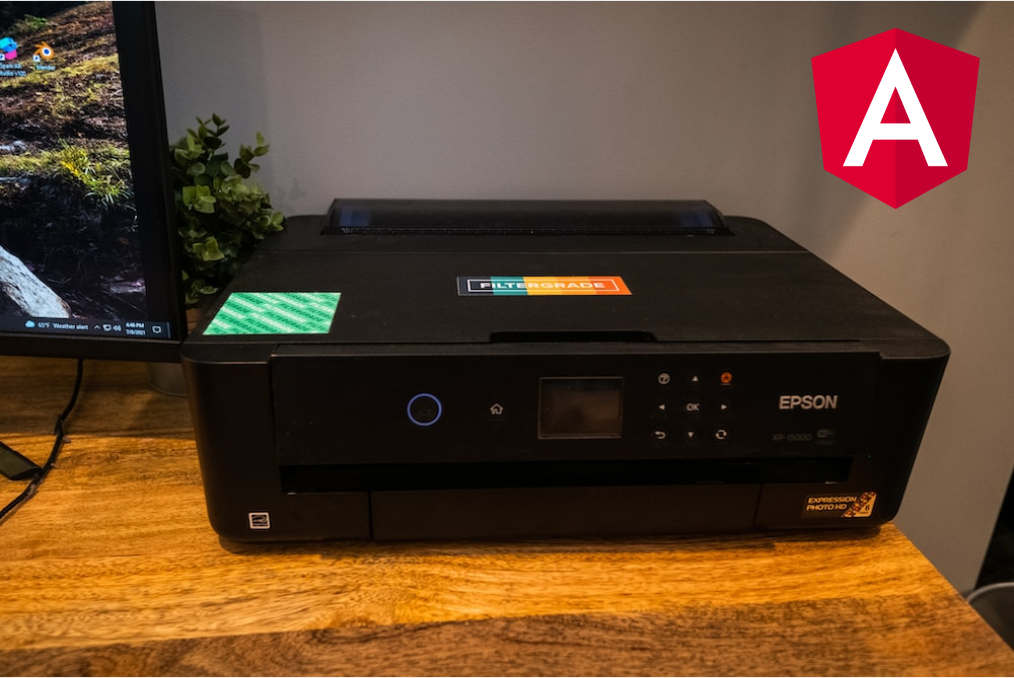
In this article, we’ll look at how to generate the Html to Pdf in an Angular project to pdf using ngx-print package.
Create an Angular application, by using following command in terminal.
ng new Print-Pdf-Angular
Type yes
to creating Angular routing and add the CSS stylesheet format.
Once the app is created, navigate to the project directory and then type the following command to open the project in the chrome.
cd Print-Pdf-Angular
ng serve
Now, You will be able to see the Angular app up and running.
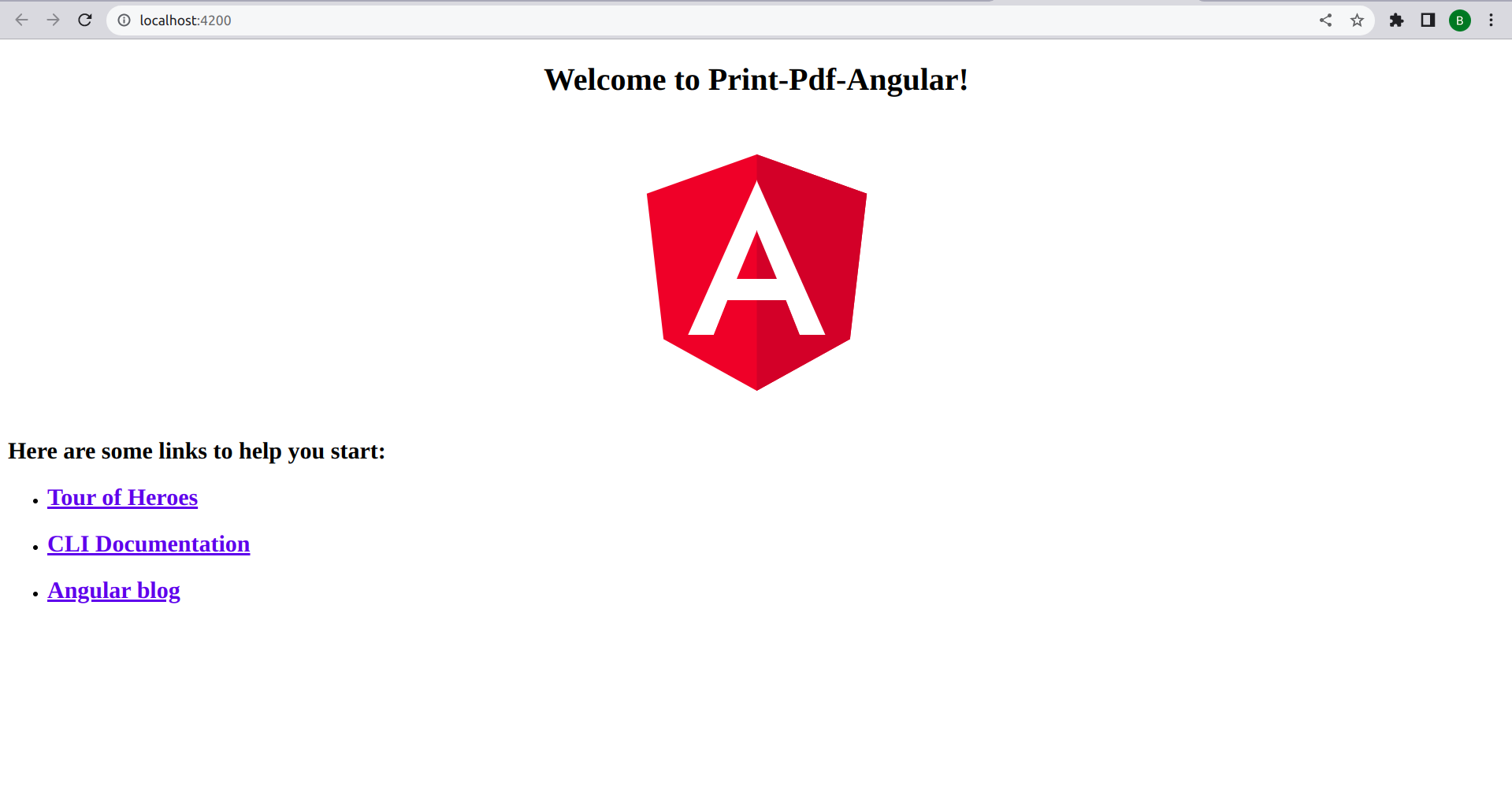
Now, lets see about Pdf conversion
First, Install following package
The npm package reference will be automatically added to the package.json file after installation.
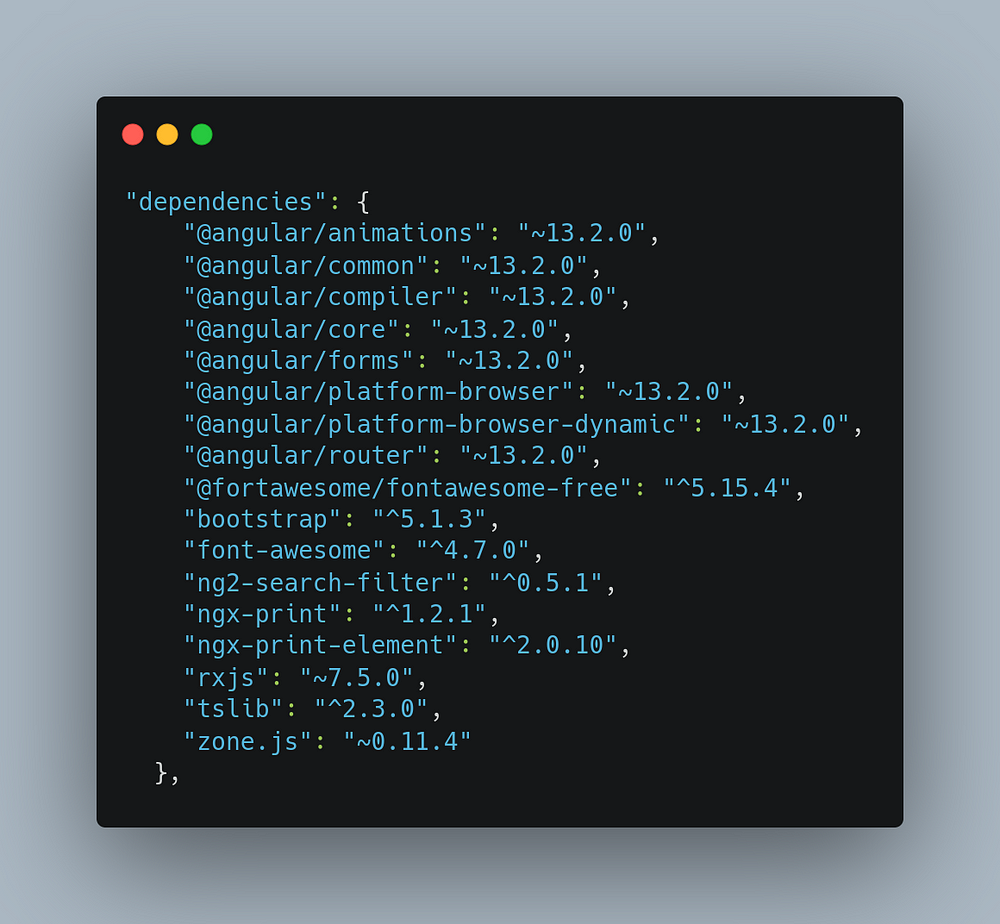
Now we need to import the ‘NgxPrintModule’ into our AppModule file to operate with the ‘ngx-library.’
import { NgModule } from '@angular/core';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { NgxPrintModule } from 'ngx-print';
@NgModule({
declarations: [ AppComponent ],
imports: [ BrowserModule, AppRoutingModule,NgxPrintModule ],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
app.component.html:
<h1>Welcome to {{ title }}!</h1>
<img width="300" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
</div>
<button printSectionId="print-section" ngxPrint>
</button>
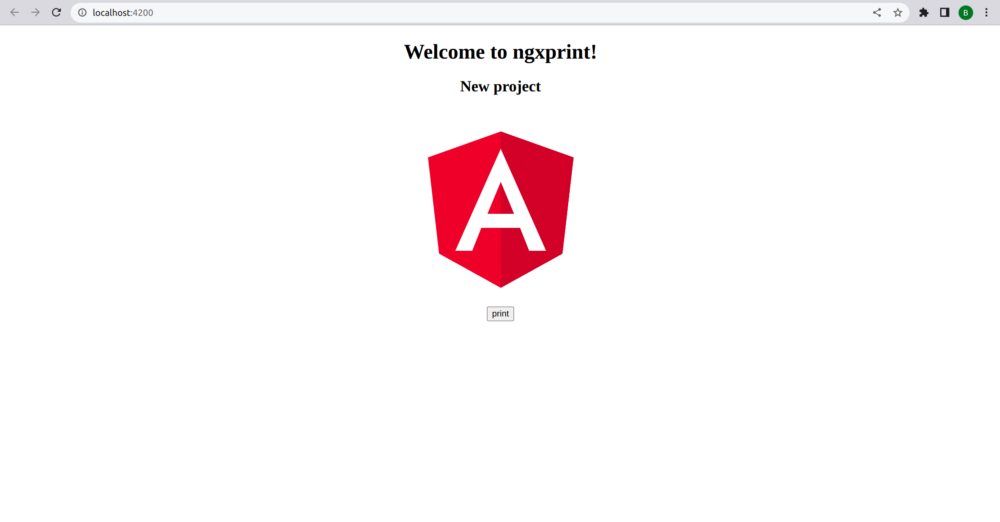
If you want to make any changes while saving the pdf add the following attribute with styles in print button. And if you change the name of the pdf to be saved add printTitle attribute.
On clicking print button the result will be updated as below.
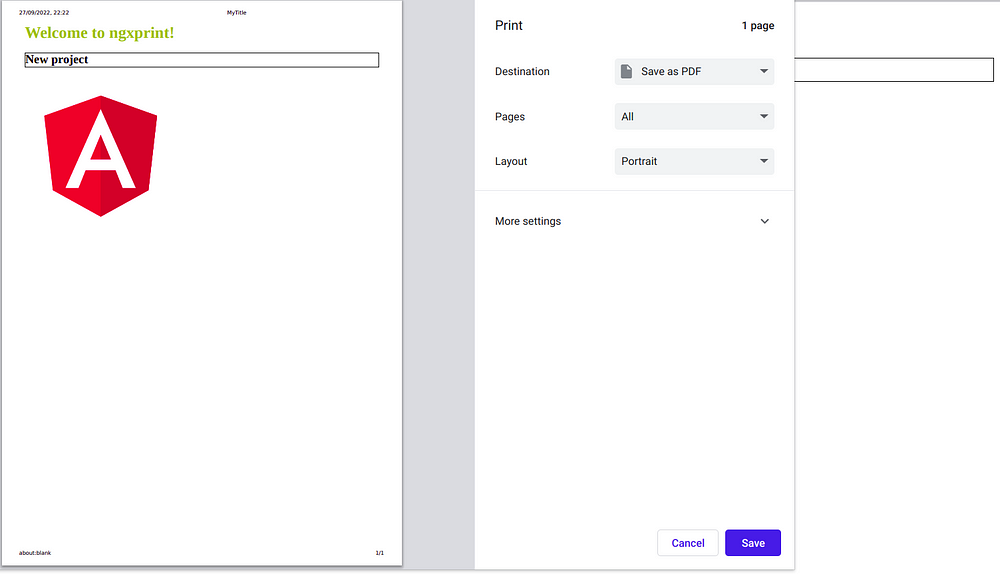
So this is the simplest and easy way to print the pdf from Angular.